Leverage the versatility of the ESP32 microcontroller to enhance your art installations with interactive, web-controlled elements. This guide is also adaptable for a range of projects, including IoT applications, smart systems, and beyond. With its robust web server capabilities, the ESP32 is an ideal solution for artists and creators looking to add interactivity and remote control to their work.
Follow this step-by-step guide to set up an ESP32 web server and bring your artistic ideas to life through dynamic, responsive installations.
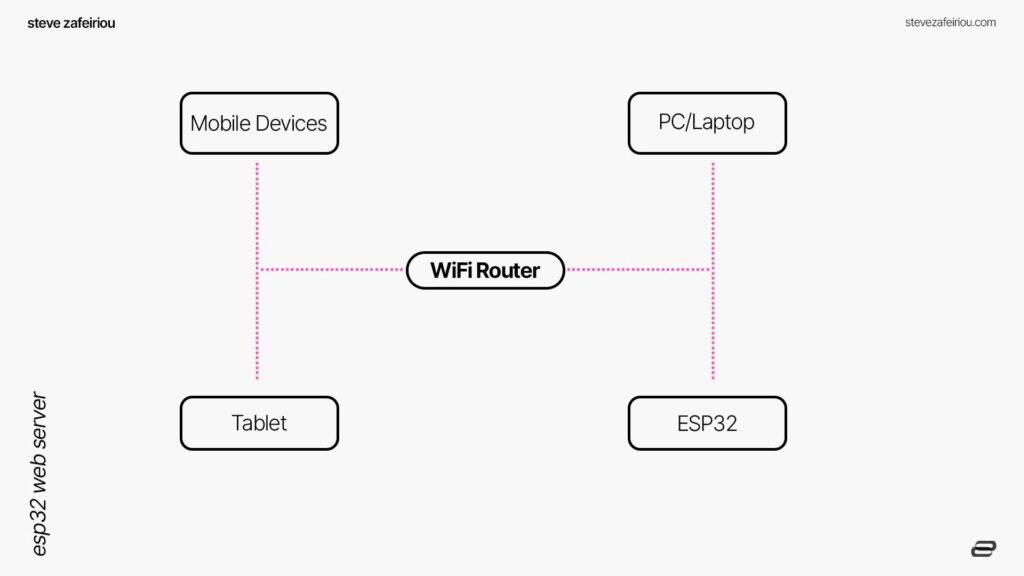
Setting up the ESP32 Web Server
Setting up an ESP32 web server can bring a modern, interactive edge to your art projects. Whether you have a concept for a digital art installation or a creative project that needs a touch of technology, this guide will help you get started with the ESP32.
Choosing the Right ESP32 Board for Your Art Project
Selecting the appropriate ESP32 board is crucial, as each type offers unique features suited to different project requirements. The ESP32 is a budget-friendly microcontroller equipped with Wi-Fi and Bluetooth, making it ideal for Internet of Things (IoT) applications.
Here are some popular options:
Board Type | Features | Ideal For |
---|---|---|
ESP32-WROOM-32 | Basic ESP32 chip | General projects |
ESP32-CAM | Built-in camera | Art needing visual input |
ESP32-S2 | Enhanced security features | Installations in public spaces |
ESP32-S3 (my favorite) | Higher performance, AI capabilities | Projects requiring AI and advanced processing |
Consider the specific needs of your project and refer to an ESP32 pinout guide if you need detailed information on the board’s pins and ports.

Installing Software and Setting Up the IDE for ESP32
To start programming the ESP32, set up the Arduino IDE as follows:

Step A: Download Arduino IDE
Get the latest version from Arduino’s official site.

Step B: Configure IDE Settings
- Open File > Preferences.
- Add this URL in the “Additional Board Manager URLs” field:
https://dl.espressif.com/dl/package_esp32_index.json
. - Click OK.

Step C: Install ESP32 Board Profiles
- Go to Tools > Board > Boards Manager.
- Search for “ESP32” and install the package from Espressif Systems.
Step D: Select Your ESP32 Board
- Go to Tools > Board and choose the appropriate ESP32 model from the list.
Don’t forget to install essential libraries, such as WiFi.h
for network connectivity and ESPAsyncWebServer.h
to manage server requests.
Configuring Your ESP32 as a Web Server
Now, let’s turn the ESP32 into a web server that can host interactive web content for your project.
Step A: Connect to Wi-Fi
Use the WiFi.h
library to set up network credentials and connect to Wi-Fi:
#include <WiFi.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
This basic configuration establishes a stable Wi-Fi connection, which is required for the ESP32 to function as a web server.
Step B: Set Up the Server
Use the ESPAsyncWebServer.h
library to set up the server.
Below is the code to initialize an asynchronous web server on port 80:
#include <ESPAsyncWebServer.h>
AsyncWebServer server(80);
void setup() {
// Wi-Fi setup here...
server.begin(); // Start the web server
}
Step C: Define Server Requests
To make the ESP32 respond to client requests, define routes that dictate how the server should respond.
Here’s a basic example:
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
AsyncWebServer server(80);
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
// Wait until the ESP32 is connected to Wi-Fi
while (WiFi.status() != WL_CONNECTED) {
delay(1000); // Wait for a second
Serial.println("Connecting...");
}
Serial.println("Connected!");
// Define a route for the web server: when the root URL ("/") is accessed with an HTTP GET request
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request) {
request->send(200, "text/plain", "Hello World"); // Send a "Hello World" message with HTTP status code 200
});
server.begin();
}
Testing Your ESP32 Web Server
Once configured, you can access the server by typing the ESP32’s IP address (found via WiFi.localIP()
) into a web browser.
The “Hello World” message will display if the setup is successful.
For additional functionality, consider incorporating features like WebSockets for real-time two-way communication, or cloud storage with Google Firebase for remote access and data logging.
Additional Considerations for Stability and Security
- Securing the Server: For public installations, it’s wise to add a password to protect the web server from unauthorized access. Implement basic HTTP authentication if the project is in a public area, ensuring that only trusted users can access the controls.
- AP Mode Setup: In addition to Station (STA) mode, where the ESP32 connects to an external network, consider setting up AP (Access Point) mode if you want the ESP32 to generate its own Wi-Fi network. This is useful if you don’t have an external Wi-Fi source, or if you want your installation to operate in a secure, isolated network.
Consider integrating sensors or actuators to make your installation responsive to motion, sound, or light, expanding the interactive potential of your project.

Programming the ESP32 Web Server for Art Control
Integrating an ESP32 web server into your art projects is like handing your art a digital remote—bringing control, interactivity, and dynamic elements to your work.
Here’s a professional overview of setting up an ESP32 web server to elevate your installations, from interactive sculptures to responsive light shows.
ESP32 Web Server Basics
The ESP32 is a cost-effective microcontroller with Wi-Fi and Bluetooth connectivity, powered by a Tensilica Xtensa LX6 processor that handles multitasking with ease.
To begin, install essential libraries such as WiFi.h
and WebServer.h
.
Once configured, the ESP32 listens for HTTP requests on port 80, allowing you to control connected devices simply by entering commands like http://192.168.1.1/ledon
in your browser.
Feature | What’s Going On |
---|---|
Microprocessor | Tensilica Xtensa LX6 |
Connectivity | Wi-Fi, Bluetooth |
Default Port | 80 |
Typical Chores | Hosting web pages, answering HTTP requests |
Read more guides on the MPU6050 gyroscope and accelerometer sensor setup or the neo 6m gps module and learn how to send real-time using websockets.
Creating Web Pages for Interactive Art Control
Transforming web pages into remote controls for your art installations is straightforward with HTML (If you’re familiar with web development, you can further customize the interface for a more tailored experience).
Design web interfaces with buttons and sliders that send requests directly to the ESP32.
Here’s a simple HTML page that allows users to toggle an LED:
<!DOCTYPE html>
<html>
<head>
<title>Art Control</title>
</head>
<body>
<h1>Control the LED</h1>
<button onclick="toggleLED()">Toggle LED</button>
<script>
function toggleLED() {
fetch('/toggleLED');
}
</script>
</body>
</html>
When the button is clicked, a request is sent to the ESP32 to toggle the LED, adding a layer of interactivity to your installation.

Integrating Sensors & Actuators for Dynamic Art
The ESP32 can interface with a wide range of sensors and actuators, enabling your art installations to respond to their environment.
For instance, you can use a motion sensor to trigger movement or a temperature sensor to create responsive displays.
Below is an example of setting up a DHT temperature sensor, where the ESP32 reads the temperature and displays it on a web page:
#include <WiFi.h>
#include <WebServer.h>
#include <DHT.h>
#define DHT_PIN 15
#define DHT_TYPE DHT11
DHT dht(DHT_PIN, DHT_TYPE); // Create a DHT object with specified pin and sensor type
WebServer server(80); // Initialize a web server on port 80 (default HTTP port)
void setup() {
Serial.begin(115200);
// Connect to Wi-Fi with provided SSID and password
WiFi.begin("your_SSID", "your_PASSWORD");
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...")
}
Serial.println("WiFi Connected");
// Set up the root URL ("/") to display the main control page
server.on("/", HTTP_GET, handleRoot);
// Set up the "/temperature" route to display the temperature reading
server.on("/temperature", HTTP_GET, handleTemperature);
server.begin();
}
void loop() {
server.handleClient(); // Handle any incoming client requests
}
// Function to handle requests to the root URL ("/")
void handleRoot() {
// Send a simple HTML page with a link to the "/temperature" route
server.send(200, "text/html", "<h1>ESP32 Art Control</h1><p><a href=\"/temperature\">Get Temperature</a></p>");
}
// Function to handle requests to the "/temperature" URL
void handleTemperature() {
float temp = dht.readTemperature(); // Read the temperature from the DHT sensor
server.send(200, "text/plain", String(temp)); // Send the temperature reading as plain text in response
}
This code enables the ESP32 to serve a webpage, read the temperature using the DHT sensor, and display it to users, creating a basic but functional interactive art control interface.
Clicking “Get Temperature” on the webpage triggers the ESP32 to read and display the current temperature from the DHT sensor.
With these tools, you can create diverse and responsive installations. For more advanced project ideas, explore my collection of arduino art projects to inspire your next interactive piece.

Connecting Art Installations to the ESP32
Integrating an ESP32 with art installations opens a world of interactive possibilities. From lighting up LED displays to controlling kinetic sculptures, the ESP32’s web server capabilities offer a versatile platform for enhancing audience engagement.
Connecting the ESP32 to Lights, Kinetic Sculptures, and More
Using an ESP32 in Station (STA) mode allows it to connect to a Wi-Fi network, obtain an IP address, and serve web pages to connected devices. For lighting effects, LED strips like WS2812B is excellent choice as it’s easily controlled by the ESP32.
For movement in kinetic sculptures, motors can be controlled with the help of motor driver modules.
Below is a quick reference table on connecting common components to the ESP32:
Component | Connection Method | Control Method |
---|---|---|
LED Strip (WS2812B) | GPIO Pin | PWM Signal |
Servo Motor | GPIO Pin | PWM Signal |
Stepper Motor | Motor Driver | Digital Signal |
Sensors (PIR, Temperature) | GPIO Pin | Analog/Digital Signal |
For detailed pin configurations, refer to my ESP32 Pinout Guide or learn about arduino lcds.
Designing a User-Friendly Web Interface for Audience Interaction
Creating a clean, accessible web interface for your art control interface involves HTML and CSS basics.
The ESP32 uses WiFi.h
and WebServer.h
libraries to manage network connections and respond to HTTP requests.
Here’s an example of an HTML page with buttons to control LED states:
<!DOCTYPE html>
<html>
<head>
<title>Art Control Interface</title>
<style>
body { text-align: center; font-family: Arial, sans-serif; }
button { font-size: 20px; padding: 10px; margin: 5px; }
</style>
</head>
<body>
<h1>Control Your Art</h1>
<button onclick="fetch('http://192.168.1.1/ledon')">Turn On LED</button>
<button onclick="fetch('http://192.168.1.1/ledoff')">Turn Off LED</button>
</body>
</html>
This interface makes it simple for audiences to interact with the installation, enhancing their experience by making the artwork responsive to their input.
Troubleshooting Art-Tech Integration Challenges
When combining art installations with ESP32, occasional technical challenges can arise.
Some common troubleshooting tips include:
- Power Check: Ensure the ESP32 has stable power, as fluctuations can disrupt connectivity.
- Code Review: Carefully review your code and connections; small errors in pin configuration or syntax can cause issues.
- Signal Strength: Monitor Wi-Fi signal strength and adjust the ESP32’s position relative to the router or other devices.
- Network Verification: Double-check SSID and password in STA mode, and confirm the ESP32 is connecting to the correct network.
If the ESP32 web server isn’t responding as expected, use the Serial Monitor for real-time diagnostics.
Incorporating the ESP32 into art installations brings digital, interactive elements to life, enhancing viewer engagement.
Starting with basic connections and gradually building complexity can unlock a range of creative possibilities that the ESP32 offers for interactive art. For more ideas, visit my ESP32 Microcontroller Interactive Art and discover the full potential of technology-driven art installations.

Check out my comprehensive guide on Arduino If Else Statements for a deeper understanding of the programming logic.
Advanced Techniques and Customization for Art Installations
When creating art installations with the ESP32, ensuring both security and functionality is essential.
Here’s a professional approach to securing and enhancing your ESP32-based installations.
Keeping Art Installations Safe and Secure
Art installations can draw considerable attention especially if installed in Public spaces, which makes securing your ESP32 web server a priority.
Here are key strategies to protect your project:
- Enable HTTPS: Secure your ESP32 communication by using HTTPS through SSL/TLS, which encrypts data and prevents unauthorized access.
- Password Protection: Add password authentication so only authorized users can access your art controls. This minimizes risks in high-traffic public spaces.
- Limit Network Access: Configure your ESP32 to operate in Access Point (AP) mode, creating a closed network for the installation. This isolated setup ensures the ESP32 communicates only with trusted devices, maintaining the installation’s integrity. For a more detailed guide, refer to our ESP32 LAN Setup page.
Enhancing Your ESP32 for Artistic Capabilities
To bring additional functionality to your ESP32 installation, consider integrating the following tools:
Library/Tool | Key Features | Use Case |
---|---|---|
ESPAsyncWebServer | Fast, asynchronous web server | For smooth, responsive web interfaces |
WebSockets | Real-time communication | Ideal for interactive installations |
Firebase Library | Cloud storage and real-time updates | Perfect for remote control and monitoring |
These add-ons allow you to build responsive web pages, enable real-time data exchange, and store or retrieve information via the cloud.
Remote Art Installations: Using the Cloud for Control and Monitoring
If your installation requires remote visibility and control, cloud platforms offer powerful solutions:
- Blynk: An accessible platform that allows you to control the ESP32 via mobile. It provides a user-friendly interface ideal for beginners or for when a professional-grade interface is required without complex coding.
- ThingSpeak: Useful for collecting and visualizing data. ThingSpeak supports HTTP-based data transmission, enabling you to monitor conditions like environmental data in real-time.
- Google Firebase: A robust cloud database for real-time updates, Firebase allows remote access to your installation settings, perfect for making adjustments on the fly.
Steps for Cloud Integration
- Select a Platform: Choose a cloud service (e.g., Blynk, ThingSpeak, Firebase) based on your project’s needs.
- Install Required Libraries: Integrate the respective cloud library in your code environment.
- Configure ESP32 to Communicate with the Cloud: Set up the ESP32 to send data to and receive commands from the chosen cloud service.
Using advanced techniques, you can make your art installations more engaging, responsive, and secure.
By securing network access, enhancing interactivity, and utilizing cloud-based control, your ESP32 art installation becomes a highly adaptable, remotely accessible work.

Creative Applications of ESP32 in Art Installations
The ESP32 is a powerful tool for artists looking to integrate technology into their artworks.
With Wi-Fi and Bluetooth capabilities, this microcontroller brings a vast range of digital possibilities to art installations, connecting the viewer and the digital world.
Case Study: “Choice” – An Interactive Sculpture Using ESP32
“Choice” is an interactive sculpture I developed that harnesses the power of the LilyGo T-Display S3 – ESP32S3 microcontroller to explore human behavior and decision-making.
Central to its concept is the idea that small, individual actions can collectively drive substantial change, an insight drawn from evolutionary theory.
In “Choice,” the ESP32 manages data from motion sensors and other inputs to dynamically create generative art in real-time, creating a generative artwork that reflects each viewer’s influence on the piece.
Project Name | Interaction Mechanism | Interaction Type | Key Components |
---|---|---|---|
Choice | Motion Detection & Web Interface | Generative Light & Movement | ESP32S3, MPU6050 Gyroscope/Accelerometer, TFT display |
Explore 9 project ideas with the Lilygo T-display S3 ESP32 microcontroller!

Core Interactive Elements
In “Choice,” the ESP32 serves as the controller for sensors, TFT display and web server, enabling real-time responsiveness to audience interaction:
- Motion Detection and Behavioral Influence: Using an MPU6050 gyroscope and accelerometer, the ESP32 captures the viewer’s movements, representing their choices in real time. The collected data influences the visual display, symbolizing how individual actions can reshape collective experience.
- Generative Art Patterns: Connected TFT display managed by the ESP32 create generative pixel art that shift with viewer interaction, resulting in a constantly evolving visual experience that adapts to each individual’s engagement.
- Remote and Web-Based Interaction: Through a web interface developed with React.js and WebSockets, viewers can engage with “Choice” on their devices, making it accessible even remotely. This connectivity, facilitated by the ESP32’s Wi-Fi capabilities, extends the reach and impact of the installation.
The ESP32 was chosen for its dual-core processing power, Wi-Fi connectivity, and compatibility with real-time data applications, all of which are critical to the project.
Operating in Station mode, the ESP32 enables stable, high-speed communication with cloud-based tools and local servers, adding an extra layer of interactivity.
“Choice” represents how art and technology can work together, and prompts viewers to reflect on their impact within shared environments.
Through this work, I highlighted how interconnectedness and behavior shape our collective experiences, using the ESP32 to demonstrate the dynamic nature of these relationships.
Ideas for Using ESP32 in Digital & Interactive Art
Here are some creative ways to bring ESP32 into your art projects:
Idea | Description | Key Components |
---|---|---|
Interactive Wall Murals | Touch/Motion responsive mural | ESP32, LED panels, Touch Sensors |
Sound-Responsive Art | Art reacts to sound | ESP32, Microphones, LEDs or Mechanical Elements |
Dynamic Sculptures | Moving sculptures | ESP32, Motors, Various Sensors |
Augmented Reality | AR + Physical art interactions | ESP32, AR glasses/devices |
Social Media-Connected Projects | Art + Social media interactions | ESP32, Network Modules, APIs |
- Interactive Wall Murals: Create murals embedded with LEDs that respond to touch or motion, displaying dynamic colors or patterns that shift as people interact with them.
- Sound-Responsive Art: Use a microphone to capture ambient sounds and translate them into light or mechanical movement, visually syncing art with music or environmental sounds.
- Dynamic Sculptures: Develop sculptures that react to environmental inputs like temperature, light, or user proximity, moving or changing shape to reflect the viewer’s presence.
- Augmented Reality (AR) Experiences: Combine ESP32 with AR technology to create interactive experiences where viewers can see digital overlays on physical objects, enriching the art experience with an additional digital layer.
- Social Media-Connected Projects: Link your installation to social media, triggering updates or live broadcasts based on user interactions, effectively turning each interaction into a social media event.
The ESP32 provides an accessible entry into the digital and interactive art world.
Whether you’re an experienced artist or exploring technology-driven art for the first time, this microcontroller can be a valuable asset for bringing interactive, digital elements to life.
Conclusion
This guide empowers artists, makers, and tech enthusiasts alike with the insights and tools to transform art installations into dynamic, web-controlled experiences.
Whether you’re looking to add interactivity, remote control, or responsive elements to your work, you’re about to change how audiences connect with your artistic practice.